I do things a bit differently, I extract all comments on the current map and then this way I'll always have an object with all notetags and nothing else. Basically, when I need the notetags from a map I'll use this function I have in my core plugin. It's an outdated function, it still works but it could use some ES6 beauty and some cleanup.
JavaScript:
function loadEventComments () {
var allEvents = $dataMap.events
var meta = {}
/* for each event load pages */
for (var e = 0; e < allEvents.length; e++) {
if (allEvents[e] === null) { continue }
var pages = allEvents[e].pages
var eventId = allEvents[e].id
var pageComments = []
/* For each page in the event, load page list*/
for (var i = 0; i < pages.length; i++) {
var page = pages[i]
var pageId = pages.indexOf(pages[i])
if (pageId <= -1) { continue }
var comments = ''
/* For each command in page list check for comments */
for (var j = 0; j < page.list.length; j++) {
var command = page.list[j]
/* if command is a comment add to comments var */
if (command.code === 108 || command.code === 408) {
comments += command.parameters[0]
pageComments.push(comments)
meta[eventId] = pageComments
}
}
}
}
return meta
}
Basically, it's a cluster F*** of for loops, looping through every event and all their pages and extracting each comment into an object sorted by event id. I can then use this object to scan through the events and check the value of the comment meta.
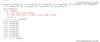
In this screenshot you can see the final result of the above method, each number is the eventId and the array is basically a single comment command per element. It's honestly my first attempt from a couple months ago so it's a bit rough.
Nonetheless, your objective here is to first loop through all events on the map, preferably when the map is first loaded, so inside DataManager.onLoad method.
JavaScript:
Alias.DataManager_onLoad = DataManager.onLoad
DataManager.onLoad = function (object) {
Alias.DataManager_onLoad.call(this, object)
if (object === $dataMap) {
// Search through all events, and their pages and extract comments.
}
}
Then it's up to you to do what you wish with these comments. I'd assume retrieving the actual meta from these comments, so I would just use this method.
JavaScript:
function getTag (text, tag) {
if (!text || !tag) { return }
var notetags = {}
var params = []
/* Setup regex and test string */
var match
var re = /<([^<>:]+)(:?)([^>]*)>/g
/* Loop exec() until all matches are found */
while (match = re.exec(text)) { // eslint-disable-line
if (!match) { return null }
/* If tag matches, & params available then push */
if (match[1].toLowerCase() === tag.toLowerCase()) {
if (match[3]) {
var args = $.toArray(match[3])
params.push(args)
notetags = params
}
}
}
if ($Utils.isEmpty(notetags)) { return }
return notetags
}
The regexp in this function is taken directly from the DataManager.extractMetadata method. What this method does is find all strings that match <tag: param parm param>
much like a plugin command. For my time control add-on plugin, the notetag <Spawn: x self all x x x x 30> will return this value when the method above is called.
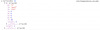
I know this probably looks like a mess lol but I hope it helps in some way. You can go about it whatever way you want, my solution works for my projects so far and you may need a different solution but the basics are all in here. Loop through events, either extract data for later or not but the last step is to search through text with regexp of your choosing and then use the data in the way you want.
P.S Just noticed you said note and not notetags, either way, the same thing really but instead of searching through each events page list you are just searching for each event note object event.note I assume. You can use my last method shown above to extract the note text as a list of arguments just like a plugin command.